Wednesday, December 24, 2008
Small Basic
It's based on .Net, and so has advanced features like auto completion and context based help. I wish I had some nice features like that when I was learning basic on my ZX Spectrum :)
Now there's a useful gift for a kid - the ability to program computers. Happy Holidays!
Edit: Note that there's also an official blog which gives updates and sample programs.
Friday, November 21, 2008
Getting fresh ideas from your staff
Having worked at several companies, I've often heard 'We want your ideas for cool new games.' If an employee suggests an idea, here's the deal they normally get:
"Give us your cool idea. If we like it, we keep all the money and you get a T-shirt. If we don't like it, you still lose all rights to it."
Sound like a good deal?
Let's say that an employee comes up with an idea you like, and it makes your company three million dollars in profit.
- Would you be willing to give that person a small percentage of the profit? (Let's say 5% - 150K).
- Let's say that someone else suggested an idea which you didn't want to use. Would you be OK saying the following? "We're not planning on using your idea now, so you can keep it".
- If the answer to these questions is yes, then you should formalize the rules and let your staff know how it works.
Inevitably, a lot of questions will come up - do my royalties continue if I leave the company? What happens if the company initially says they do not want my idea, but use it later on? What happens if sequels are made? What if my idea is smaller, like a mini game or new funky shader? etc.
Here I have a few suggestions:
- Answer all these questions on an intranet page which people can look at without having to ask the questions themselves. If people have to ask, you put them in the awkward situation of implying "I've got a cool idea, but I don't want to give it to you."
- Let your guiding principle be that you want to be nice to the employees, so they're happy to share. They wont share if they don't trust your intentions.
- Your intranet page explaining how the deal works is basically a sales letter, or offer. If you don't make the offer tempting enough, they'll just hang on to their ideas.
- You can cover your back with whatever legalese you want. Just make your intentions clear and honest, and 'do the right thing' if issues arise.
It would take some courage to formalize something like this, but it's my feeling that a company capable of doing so is going to be making a lot more money.
Saturday, November 08, 2008
Free Subversion hosting at XP-Dev.com
I decided to look for another alternative and found this list of free SVN hosting sites. From the list I decided to choose XP-Dev.com. It's a relatively no frills site, but free. It's only been a couple of days so far, but I really like it. The guy who runs it is very friendly and quick to help.
If you're thinking of moving from Assembla to XP-Dev.com, here are some steps which make it easy:
- Check all your code in to Assembla.
- Download a dump of your repository from Assembla. To do this, click on: SVN/Trac > Import or Export your Subversion repository here > Get SVN Backup
- Set up an account and repository with XP-Dev. This takes about a minute, it's easy. Note that your username and repository name together become the url of your repo, e.g. BradPitt_MyRepoName
- Email your Assembla repository dump and new repository name to XP-Dev support, and they'll set it up for you.
Then just set up a new folder to point to your repository. (With TortoiseSVN - Make a folder. Right click and select 'SVN Checkout'. Give it the server URL and username/password as needed.) Also I recommend keeping your old Assembla linked folder around, just in case you forgot to check a file in.
Happy coding!
Thursday, July 24, 2008
Free 3D Modeling Packages
Here's a list of free 3D modelling tools which could be used in developing your own games:
- Blender
- Sketchup (mentioned in an earlier post)
- trueSpace
I'll also give a mention to Milkshape, which is a $35 shareware modeling program with a 30 day free trial.
Friday, July 18, 2008
How to compile from the command line with Visual Studio
I often forget how to compile a project from the command line. Here's my little reminder:
- First run C:\Program Files\Microsoft Visual Studio 8\VC\vcvarsall.bat
- Then call something of the form: devenv c:\Foo\MySolution.sln /build "Release"
Note that this works for Visual Studio 2005. Small changes may be needed in other versions. Also, I don't think 'devenv' comes with the Express edition - try using 'VCExpress.exe' instead.
Sunday, July 13, 2008
TrueType to bitmap conversion
A friend of mine has written a shareware app called Fontrast which does just this. Well worth the $15 compared with spending the time to write it yourself...
Tuesday, July 01, 2008
List of Game Middleware
Thursday, June 26, 2008
What does Cuda code look like?
A couple of posts ago I gave an overview of what Cuda is. Dr Dobbs has a great series of articles introducing Cuda. In this post, I'm going to blatantly pinch a bit of their code, to show what Cuda looks like.
Let's say we have an array of floats, and we want to increment each element in the array by 1. The regular C code for this might look like so:
void incrementArrayOnCPU(float *a, int N)
{
for (int i=0; i < N; i++)
{
a[i] = a[i]+1.f;
}
}
With Cuda we can instead run many threads at once with each incrementing one element in the array.
The function which runs on the GPU is called the 'Kernel'. The kernel can't access regular memory, so any memory it uses needs to be specially allocated. Look at the following code (which is modified from the Dr Dobbs sample for readability, and may not compile now).
void IncrementArray()
{
// Create an array of 102 floats
#define N 102
float mainMemoryArr[N];
for (i=0; i<N; i++)
mainMemoryArr[i] = (float)i;
// Copy data to the graphics card memory
float *gpuMemoryArr;
cudaMalloc((void **) &gpuMemoryArr, size);
cudaMemcpy(gpuMemoryArr, mainMemoryArr, sizeof(float)*N, cudaMemcpyHostToDevice);
// Calculate how many 'blocks' of threads we'll run
int blockSize = 4;
int nBlocks = N/blockSize + (N%blockSize == 0?0:1);
// Run the GPU function
incrementArrayOnGPU <<< nBlocks, blockSize >>> (gpuMemoryArr, N);
// Copy the modified memory back to main memory
cudaMemcpy(mainMemoryArr, gpuMemoryArr, sizeof(float)*N, cudaMemcpyDeviceToHost);
}
So the code above does the following:
- Allocates memory on the graphics card.
- Copies the array there.
- Runs 26 'blocks' of 4 threads each. (A total of 104 kernel executions)
- Copies the modified memory back to main memory (which automatically blocks on the kernel threads completing).
The 'kernel' function which runs on the graphics card looks like this:
__global__ void incrementArrayOnGPU(float *arr, int N)
{
int idx = blockIdx.x*blockDim.x + threadIdx.x;
if (idx<N)
arr[idx] = arr[idx]+1.f;
}
So it looks like C, apart from a couple of small differences and notes:
- The keyword __global__ identifies the function as a kernel function which will execute on the GPU.
- There are 'blockIdx', 'blockDim' and 'threadIdx' variables, which are used to calculate the 'index' of this thread. I'll not go into the details of what exactly they mean, but it's pretty simple.
- The 'if(idx<N)' part is because the kernel will actually be called for slightly more than the number of threads we request.
The 'blocks' construct seems like a bit of nuisance. It exists because that's the way the hardware works. I believe you could specify a block size of 1 to simplify things, but the reality would be that you'd have several of the processing elements sitting idle. You need to experiment with different block sizes to find which gives your program the best performance.
This was a trivial example of what can be done with Cuda. In reality, the kernel function would likely be doing quite a bit more work. Overall, if you're familiar with C, I think you can see that programming with Cuda is not too strange. There's just a couple of extra keywords and functions to call, but it's quite easy to understand...
If you want to learn more, start by reading the Dr Dobbs articles, and downloading the SDK from the Cuda Zone. The SDK comes with a load of samples for you to prod at. You can run in software emulation mode if you don't have a swanky graphics card, but it's pretty slow.
Introduction to OpenMP - Easy multi-threading for C/C++
Parallel allows you to specify a block of code which executes multiple times in parallel:
void ParallelTest()
{
#pragma omp parallel num_threads(4)
{
int i = omp_get_thread_num();
printf_s("ParallelTest: Hello from thread %d\n", i);
}
}
Results in the output:ParallelTest: Hello from thread 0
ParallelTest: Hello from thread 3
ParallelTest: Hello from thread 1
ParallelTest: Hello from thread 2
The ‘for’ directive allows a for loop to be split up and executed in several threads:
void ForTest() { #pragma omp parallel for for (int j=0; j<=4; ++j) { int threadNum = omp_get_thread_num(); printf_s("ForTest: Hello from thread %d - loop iteration %d\n", threadNum, j); } }Results in the output:
ForTest: Hello from thread 0 - loop iteration 0
ForTest: Hello from thread 2 - loop iteration 3
ForTest: Hello from thread 1 - loop iteration 2
ForTest: Hello from thread 3 - loop iteration 4
ForTest: Hello from thread 0 - loop iteration 1
The thing which I find really cool about OpenMP is that it requires such little change to the code. Normally you’d need to write a lot of lines of code to accomplish the same results we see above, but with OpenMP it’s often as little as a single line – which you can comment out to go back to a single threaded approach. So if you find an inner loop which is hogging performance, and lends itself to parallelization, you can quickly make it multithreaded. It's supported on Xbox 360, but not across all game consoles. It's certainly useful for speeding up your tools.
For a list of other compilers which support OpenMP, see here. Note that Visual Studio 2005 does support it, but isn’t listed, as they just list the latest version.
To enable OpenMP in your Visual Studio C++ project, you need to:
- #include <omp.h> // Include OpenMP
- Go to your ‘Project properties->C/C++->Language->OpenMP Support’, and set it to ‘Yes’.
In my previous post, I introduced Cuda. These two technologies are very different - OpenMP requires minimal code change and runs on CPU, while Cuda requires much more work but allows you to run on the GPU with many more cores.
Tuesday, June 24, 2008
What is Cuda?
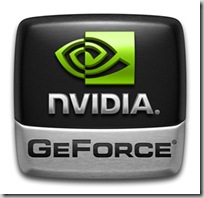
I recently took a look into Cuda. It's a technology from nVidia which allows you to run C code on the GPU of the GeForce 8 series and above.
The cool thing is that the top end nVidia card currently has 240 processing units, and you can put a couple in one machine. So for perhaps around a couple of grand, you can get a 480 core PC - kinda. You don't have to spend that much though; at the time of writing, a 128 processor GeForce 9800 is $200, and a 32 processor 8800 is only $70.
So the promise is that you can write bits of C code, and then execute the same code many times in parallel on the graphics card, beating how long it would take on CPU.
The Cuda website lists almost a hundred projects which have used Cuda, with performance improvements ranging from 2x to 240x. The main reason for the big range is that Cuda's not good for everything. If the piece of code gets large, uses a lot of memory, contains branching and random/scattered memory access, then performance goes down. The highest performance is for short programs with little branching and little memory access.
Some problems are inherently more suited to Cuda-fying than others, but the programmer can still make a big difference by writing their code with the hardware in mind. Working at a low level like this, learning the hardware, and optimizing code to run as efficiently as possible on it, is very similar to the kind of skills used when coding for high performance on games systems, and in particular to PS3 Cell programming. So if you're looking to get into games, then impressing the programmers who interview you with your Cuda skills would be a great way to get your foot in the door!
Thursday, June 05, 2008
New Wall-e Trailer
Here's a fun trailer which introduces some of the robots from the film.
Monday, June 02, 2008
Free Visual Studio Professional - for Students
Hmm, looks like I've found another use for the interns... :)
Friday, May 30, 2008
How to Evaluate Middleware
Most commercial games have got some middleware in them somewhere. Some types of middleware include video players, sound systems, physics systems, UI systems. I've already mentioned a few of the pro's and con's of using middleware in a previous post.
You need to be careful when choosing middleware. If you rush in, you could choose a system that doesn't do what you need, costs more than you need to pay, or gives you some nasty surprises down the road.
Here's a breakdown of some recommended steps in choosing middleware. Some are obvious, but some are less so.
- Write a list of all the things you want the middleware to do, or be used for.
- Do some searching and find all the different options which exist. Don't dig into the details of any of them just yet, just find out all your options so you've got the best chance of finding a good fit.
- Ask other game programmers at your studio if they've used any of the systems in the past. If you have sister studios, then ask them too, and also see if they have hand written solutions which you could reuse.
- Make a comparison spreadsheet of the high level features of each option, how well it meets your needs, and the pricing.
- If you are developing for consoles, you likely need to be more concerned by how the engine component behaves. Ask whether you can override file access and memory allocation. Also ask if they use STL, or anything like it, and what their stance is on allocating memory at run time.
- If your game is going to be localized, and the middleware will be using localized assets, work out how it will handle the different languages. E.g. Can the movie player handle different language tracks? Will the sound tool help you find wav files which haven't been translated? Will the UI support the large character sets needed for Japanese and Korean?
- Consider how much of an evaluation you can do without coding. Integrating middleware at a code level takes a long time. Can you evaluate tools first?
- Pick one or two options to evaluate in a hands-on fashion, and check with your boss and your legal advisor that they're OK with the concept of you using it, the price, and the license terms.
- Integrate it with your engine in a code branch. Don't start relying on it yet though. It's easy to slip into assuming that you're using it.
- Create a stress test to push it to its limits.
- Check that it can do everything you need it to (to a reasonable degree of accuracy).
- If you're focused on such things, check the memory allocations made, performance, and amount of memory used.
- Have a review meeting in which you go over your findings and decide whether to officially approve the system for use.
- If approved, then you can integrate your code branch to the main branch, and encourage people to start using it.
If you've used middleware before, it's fun to look at the above list and see the mistakes you made in the past :)
Monday, May 26, 2008
Game Development Studio Map
Is your studio there? If not, you can add it, or add a post and I'll add it for you.
View Larger Map
Saturday, May 24, 2008
Game Programming Books for Beginners
Pick a Language
First of all, you need to understand that there are several many different programming languages. You need to pick a programming language first, and then pick a book which focuses on it. The language will either be in the title of the book, or will be mentioned in the blurb on the back. So which language should you pick?
- C / C++As I've said in a previous article, if you're looking to get a job in console game development, then you should focus on 'C++'. As programming languages go, it can be pretty tricky for beginners. If you've done some programming before, or are looking at applying for a games job in a few years, then try C++.
- C#C# is a relatively new language which Microsoft created. It's similar to C++ in how it looks, but it's quite a bit simpler to use. If C++ sounds a bit daunting, and you'd like to see your game working sooner, I'd start with C#. After you're comfortable with C#, you can come back to C++ later.
- JavaJava is somewhat similar to C# from a language perspective. Some types of commercial games (e.g. cell phone) can be written in Java. I'm generally not very familiar with it though, and tend to recommend C# over it if you're thinking of getting into console development.
- Basic
There are many different types of Basic. Basic is generally seen as the easiest type of language to get started with, though personally I think that it's not going to be significantly easier than C#, and C# is going to get you closer to C++. If you're just looking to have fun though, and take your first steps in programming, there's nothing wrong with Basic. I first learned to program on Basic (on the ZX Spectrum), and it kept me busy coding for years.
Publishing DateThe next thing to look for is how old the book is. New versions of programming languages, tools, and libraries (don't worry if you don't know what I mean) come out all the time, and it's best to start by learning with the latest stuff. Aim to find a book that's been updated in the last five years.
Skill LevelFinally you need to pick the skill level. It's safest to aim low if you're just getting started. I recommend seeing if the index for the first couple of chapters has sections for things like 'variables', 'the "if" statement', 'for loops, while loops', and perhaps a little later 'arrays'. The words might be slightly different. Looking at the index and reading the blurb on the back should give you an idea of whether the book is for you.
Borrow or Buy?Programming books can be pricey. I recommend getting started by using library books or books available for free online. Then if you like the book, and feel like you want to keep on coding, you can buy it later.
Google has many books online. It might not be the best way to read a book if you're programming, but it's certainly good for seeing if a book is for you.
Is it any good?Read the user reviews for the book on Amazon.com. Remember that skill level might come into play - someone might say a book is bad because it was too simple, but that might be just what you're after.
"CD Included"
These books will usually come with a CD/DVD which contains 'all you need to get started'. It's fine to use the contents of the disk, but if you get the book without the disk, then note that the tools on the disk are usually freely available online.
Example Books
I did a quick search for example books in each category. The links to most of the books show the full contents of the book.
- C++
- Beginning C++ Game Programming by Michael Dawson
Note how the second and third chapters have sections on 'if', 'while', 'loops' and 'arrays'. This looks like a good book for absolute beginers. - C#
- Basic
- Beginner's guide to DarkBASIC game programming
- Sams teach yourself game programming with Visual Basic in 21 days
- Game programming for teens (this book focusses on Blitz Basic)
Thursday, May 15, 2008
List of Automated Build Systems
Once you've got a game in development, it becomes important to streamline your build process, so you can quickly create a build without a lot of manual work. (I explain what a build process is at the bottom.)
This is a list of products I've found which aim to speed up and automate the compilation and build process.
- AnthillPro3
- Automated Build Studio
- Bamboo
- CruiseControl.NET
- Electric Cloud
- FinalBuilder
- HiveBuild
- Incredibuild
- MSBuild (part of Visual Studio)
- Nant
- Parabuild
- TeamCity
- Visual Build
- Zutubi
What do you use?
What's a build process?
A typical build process might look like this:
- Fetch the latest code and data from source control.
- Run a perl script which updates a version number in a header file.
- Compile the game, and the editor.
- Run the editor in command line mode, telling it to 'pack' all the game data for the various levels of the game.
- Run a script which packages the compiled game and game data into a windows installer 'MSI' file.
- Copy the MSI to the network for consumption by the team.
- Send an email to the team, saying that the new build is available.
The reality is that they get a lot more complicated than this, as some steps may fail, you might be building for multiple consoles, you might be building from multiple code branches, you might have to build for multiple languages, etc...
Components of a Console Game
Something that comes up from time to time is the need for a full breakdown of everything which makes up a game. It's a tricky thing to do, as each game is different, and there are many approaches to any given part. Here's a quick brainstormed list though, to give an idea of what goes into a game.
- Engine
- You can choose to license/use an existing engine, or build your own. There are pro's and con's to both approaches.
- The Basics
- Memory allocation
- Debug text output to screen and debugger
- File IO
- Reading config / xml files
- Build process
- Rendering
- Shader pipeline
- Render opaque static objects
- Render skinned objects
- Render transparent objects
- More complex material effects. Lighting, environment mapping, bump/parallax, etc.
- Screen effects. HDR/Bloom, lens flare, depth of field etc.
- Animation
- Hierarchical skinned animation, including art tool exporter and pack process.
- Blending of animations
- ‘Blend shape’ vertex animation
- Ability to modify animated meshes ‘by hand’ (e.g. IK or head tracking).
- Events - the ability to send events between systems/objects.
- Gameplay
- Player
- Player moves
- Walk / run / jump
- Attack moves
- Swim
- Pick up weapon
- Behaviour
- Rag doll
- IK for foot placement
- Look at interesting objects
- Conversations
- Attached physics objects / cloth
- Player moves
- NPC
- Ambient characters / animals
- Enemies
- Bosses
- Systems
- State machine (often hierarchical).
- Attaching of FX/sound.
- Route planning.
- Method for spawning / handling high numbers.
- Gameplay Widgets
- Static object
- Breakable physics objects
- Weapons
- Treasure chest
- Collectables
- Pushable objects
- Doors
- Vehicles
- Non-Gameplay Widgets
- Butterflies.
- Player
- Networking / Multiplayer
- Guaranteed / Non guaranteed messaging system.
- Synchronization strategy.
- Testing harness.
- Tools (some mentioned in other points)
- Art pipeline for objects, environment and characters.
- Level building tool for level layout and scripting.
- UI building tool.
- Systems
- UI
- Tool for building and animating UI, Scripting system for ‘gluing it together’, Integration with game engine.
- Runtime component for playing the UI.
- Font rendering.
- Localization system.
- Sound
- Loading sounds, Memory resident sounds, Streamed sounds, 3D position support, Effects (e.g. Echo), Categorization of sounds (game/dialogue/menu), Volume controls by category, Connecting of sounds to other widgets.
- Physics
- Select from free or purchased middleware and integrate with engine.
- FMV
- Select from free or purchased middleware and integrate with engine.
- Cinematics
- If game calls for conveying story, build a system for using the game engine to enact story sections.
- FX
- Particles, Ribbons, Shrapnel, Lights, Water, Fire, Fog, Rain, Tool for artist to configure effects, Tool for adding effects to cinematics.
- Networking / Lobby
- UI
- UI Screens
- Front end
- Basic: Attract mode, Main Menu, Level Select, Load/Save, Options, Cheats, Unlocked Content, Credits.
- Network play related: Chat room, Matchmaking, Leaderboard, Clans, News.
- In game pause menu
- Map, Objectives, Inventory, Some repeated functionality from front end.
- TRC Compliance
- Achievement points
- Front end
- Infrastructure
- Automated building and testing
- Builds / Fire fighting
- Automated error reporting
- ‘Crash dump’ information per platform, Detailed error reports sent by email automatically when tools fail.
Thursday, April 24, 2008
Dr Dobbs Game Challenge - Win Cash!

Dr Dobbs Magazine has teamed up with Microsoft for a game programming competition. You modify a game they give you (using a trial version of Visual Studio 2008), and stand a chance of winning part of a $10,000 cash prize pool.
Even if you don't win, this kind of stuff is great on your resume and good for your brain. It shows that you're eager and competent, and gives you a demo you can show to people.
It's likely C# based, which isn't what I'd generally recommend focusing on, but it's a useful language to know.
Friday, April 11, 2008
List of UI systems for games
Commercial Systems
- Anark
- Scaleform Gfx
- Menus Master
- XUI (360 only - installed as part of Xbox 360 SDK)
- CEGUI (Crazy Eddie's GUI) (Free and can likely be easily ported to console)
Am I missing any? Post the name and I'll add it to the list (and not publish the post, to keep the page cleaner).
Sunday, March 02, 2008
Can't select Debug or Release configurations in Visual Studio 2005?
- Select 'Tools->Options' and if it's not already checked, check 'Show all settings'.
- Now choose 'Projects and Solutions' and check 'Show advanced build configurations'.
Perhaps now that I've blogged it, I'll remember it next time... :)
Saturday, February 16, 2008
OpenSVN Review
UPDATE #2: Assembla is no longer free, so I looked for other options and I've switched to XP-Dev.com.
UPDATE #1: Following writing this review, and the suggestion in the posts below, I looked into using Assembla. I've now been using it for several months for version control for home. I find it very fast, and haven't seen it go down once. I highly recommend it!
ORIGINAL POST:
I recently bought a laptop, so that I don't have to be sat at a desk to ruin my eyes. I wanted to use my laptop for a bit of coding, but Perforce, the version control system I had been using, was installed on my desktop machine. This meant that my desktop had to be turned on, for my laptop to be able to check things in and out. This bugged me, so I started to look for solutions were my source could be hosted online.
There are lots of companies out there who will host your source code. They mostly use the open source systems CVS and Subversion (also known as SVN). Some companies charge monthly, with increasing fees for increasing server space and/or numbers of users. Some have a 'free' service, but the restrictions are pretty tight. If you're interested in open source development, and don't mind the world seeing your code, then there are other free hosting options out there too.
I found one SVN hosting site which was completely free, didn't have tight restrictions, and didn't require that your code is open source - OpenSVN (OpenSVN.csie.org). It's operated by some students at National Taiwan University. As I'm always interested in a bargain, I thought I'd give it a try. After 5 weeks of using it, here are my thoughts:
- The presentation on the OpenSVN site is poor. The other project hosting sites, with their art work and stock photos of people pointing at monitors, make you feel a lot more comfortable about putting your valuable source in their hands. Plus the OpenSVN site has some kind of bad certificate, which makes IE7 give dire warnings.
- After the initial setup of the account, you don't need to go back to their site. Just use TortoiseSVN (or a similar windows client) to manipulate your files. I chose TortoiseSVN, and after a short learning period, I really like it.
- The server has been down on four days of the 5 weeks that I've been using it. This hasn't caused big problems for me, as I just code on my own, for fun, and am OK waiting for them to fix it. If however I was working in a group, at a serious development company, this level of down-time would be unnacceptable.
- I've not needed tech support, but doubt that whatever they provide would be on a par with what a professional hosting company could provide.
- Initially I was a bit concerned about whether it would be too slow. I've found it to be fast enough for my needs - just checking in/out relatively small amounts of code. I'd say it takes about 15 seconds to either check in, or get latest, for an average amount of change. If however you were thinking of storing larger binary data (e.g. textures) then it may be worse.
Overall, OpenSVN is OK. I find the occasional down time acceptable, considering that it's free. If you use it, I recommend that you also make your own backup procedures, and not rely on theirs. For home development, when it comes to backing up a version control system, I'm of the belief that it's OK to just back up the last good version. If the history gets lost, then it's just tough turkey.
If you're new to SVN and hosted version control, then it's a good idea to use OpenSVN first so you can get a better feel for what your needs are before shopping for a commercial/reliable service.
What do you use?
Friday, February 01, 2008
Version Control at Home
Basically a version control system is a repository which stores a history of all the major versions of your source files. You can modify a bunch of files, and then 'check them in' together. Since the system knows all the versions of your files, you can do things like:
- Compare different versions of a file, to see what changed, and when code was introduced.
- 'Get' and old version of all your source. This can be handy if your code stops working, and you have trouble working out why. You can go back, find the version which last worked, and then compare the versions.
- Discard the changes you've made since you last checked in, reverting back to the last good version. This is one of my favourites :)
CVS and Subversion are both free. Perforce is free for small scale personal use (2 users max). Perforce is quite popular in gaming companies too. I use Perforce at work, and so decided to use it at home too. Installing a version control system can be a bit daunting, but there are good tutorials out there if you look.
I don't recommend version control to the absolute beginner, it's better to focus on learning the language and making little sample apps. But if you're going to work on one program, for a while, then I recommend it.
Friday, January 25, 2008
Gateway T5450 Laptop M-6750 Review

Pros:
Cons:
Overall:
{Edit - Wow, this post got a lot of hits. It seems some people have had a lot of problems with the machine. Mine's still fine 7 months on, but buyer beware...}